创建视图
<?php /* @var $this yii\web\View */ use yii\helpers\Html; $this->title = 'About'; $this->params['breadcrumbs'][] = $this->title; ?> <div class="site-about"> <h1><?= Html::encode($this->title) ?></h1> <p> This is the About page. You may modify the following file to customize its content: </p> <code><?= __FILE__ ?></code> </div>
进行编码和/或为了过滤从最终用户来的数据以避免XSS攻击。应该通过调用 yii\helpers\Html::encode() 编码纯文本,
以及调用 yii\helpers\HtmlPurifier 过滤HTML内容。
<?php /* @var $this yii\web\View */ use yii\helpers\Html; use yii\helpers\HtmlPurifier; $this->title = '关于我们'; $this->params['breadcrumbs'][] = $this->title; ?> <div class="site-about"> <h1><?= Html::encode($this->title) ?></h1> <p> This is the About page. You may modify the following file to customize its content: </p> <p> <?= Html::encode("<script>alert('alert!');</script><h1>ENCODE EXAMPLE</h1>>") ?> </p> <p> <?= HtmlPurifier::process("<script>alert('alert!');</script><h1> HtmlPurifier EXAMPLE</h1>") ?> </p> <code><?= __FILE__ ?></code> </div>
请注意,Html::encode()函数中的JavaScript代码过滤后显示为纯文本。HtmlPurifier::process()调用后,只有h1标签显示。
-
视图是由控制器提供的,应该放在@app/views/controllerID文件夹中。
-
视图是一个小窗口渲染呈现,应放入 widgetPad/ views 文件夹。
-
render() − 渲染一个视图,并应用布局
-
renderPartial() − 渲染视图,但不使用布局
-
renderAjax() − 渲染视图但不使用布局,但所有的注入JS和CSS文件
-
renderFile() − 在一个给定的文件路径或别名来渲染视图
-
renderContent() − 渲染一个静态字符串并应用布局
-
render() − 渲染一个视图。
-
renderAjax() − 渲染视图但不使用布局,但所有的注入JS和CSS文件。
-
renderFile() − 在一个给定的文件路径或别名来渲染视图。
第4步 - 在 views/site 文件夹里边,创建两个视图文件: _view1.php 和 _view2.php
_view1.php −
<h1>这是视图 - _view1.php 的内容</h1>
_view2.php −
<h1>这是视图 - _view2.php 的内容</h1>
<?php /* @var $this yii\web\View */ use yii\helpers\Html; $this->title = '关于我们'; $this->params['breadcrumbs'][] = $this->title; ?> <div class="site-about"> <h1><?= Html::encode($this->title) ?></h1> <p> This is the About page. You may modify the following file to customize its content: </p> <?= $this->render("_view1") ?> <?= $this->render("_view2") ?> <code><?= __FILE__ ?></code> </div>
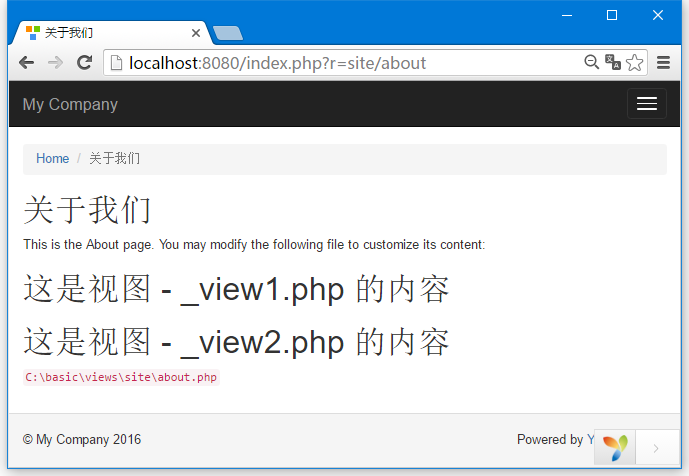
当渲染视图,可以使用视图名称或视图文件的路径/别名来定义视图。视图名称解析按以下方式 -
-
视图名可以省略扩展名。例如,about 对应于 about.php 文件。
-
如果视图名称开头“/”,那么,如果当前活动的模块是forum,视图名为comment/post,路径将是 @app/modules/forum/views/comment/post。如果没有活动的模块,路径将是@app/views/comment/post。
-
如果视图名称以“//”开头,对应的路径是@app/views/ViewName。例如,//site/contact 对应于@app/views/site/contact.php
-
如果视图名称是contact,并在上下文控制器是 SiteController,那么路径将是 @app/views/site/contact.php。
-
如果 price 视图要在 goods 视图中渲染,那么,如果它在 @app/views/invoice/goods.php 渲染,那么 price 会被解析为 @app/views/invoice/price.php 。
在视图中访问数据
public function actionAbout() { $email = "admin@xuhuhu.com"; $phone = "13800138000"; return $this->render('about',[ 'email' => $email, 'phone' => $phone ]); }
<?php /* @var $this yii\web\View */ use yii\helpers\Html; $this->title = '关于我们'; $this->params['breadcrumbs'][] = $this->title; ?> <div class = "site-about"> <h1><?= Html::encode($this->title) ?></h1> <p> This is the About page. You may modify the following file to customize its content: </p> <p> <b>email:</b> <?= $email ?> </p> <p> <b>phone:</b> <?= $phone ?> </p> <code><?= __FILE__ ?></code> </div>
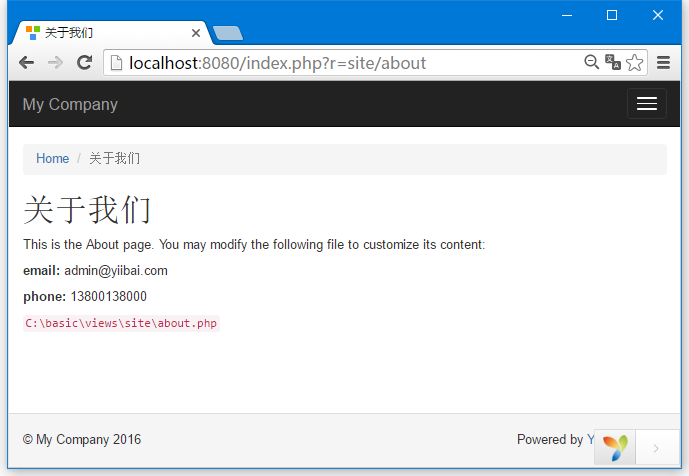