Angular 2 JavaScript 環境配置
本章節我們為大家介紹如何配置 Angular 2 的執行環境。
本章節使用的是 JavaScript 來創建 Angular 的應用,當然你也可以使用 TypeScript 和 Dart 來創建 Angular 應用 。
本章節使用到的檔目錄結構如下所示:
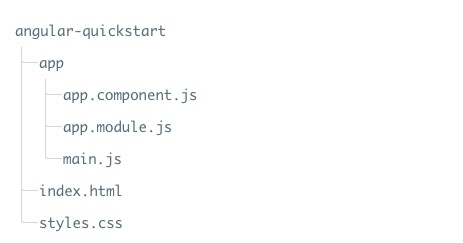
創建配置檔
創建目錄
$ mkdir angular-quickstart
$ cd angular-quickstart
載入需要的庫
這裏我們推薦使用 npm 來作為包的管理工具,如果你還沒安裝npm或者不了解 npm 可以查看我們的教學:NPM 使用介紹。
創建 package.json 檔,代碼如下所示:
package.json 檔:
{
"name": "angular2-quickstart",
"version": "1.0.0",
"scripts": {
"start": "npm run lite",
"lite": "lite-server"
},
"license": "ISC",
"dependencies": {
"@angular/common": "2.0.0",
"@angular/compiler": "2.0.0",
"@angular/core": "2.0.0",
"@angular/forms": "2.0.0",
"@angular/http": "2.0.0",
"@angular/platform-browser": "2.0.0",
"@angular/platform-browser-dynamic": "2.0.0",
"@angular/router": "3.0.0",
"@angular/upgrade": "2.0.0",
"core-js": "^2.4.1",
"reflect-metadata": "^0.1.3",
"rxjs": "5.0.0-beta.12",
"zone.js": "^0.6.23",
"angular2-in-memory-web-api": "0.0.20",
"bootstrap": "^3.3.6"
},
"devDependencies": {
"concurrently": "^2.0.0",
"lite-server": "^2.2.0"
}
}
由於 npm 官網鏡像國內訪問太慢,這裏我使用了淘寶的npm鏡像,安裝方法如下:
$ npm install -g cnpm --registry=https://registry.npm.taobao.org
執行後我們就可以使用 cnpm 命令來安裝模組:
$ cnpm install
執行成功後,angular-quickstart 目錄下就會生成一個 node_modules 目錄,這裏包含了我們這個實例需要的模組。
創建 Angular 組件
組件(Component)是構成 Angular 應用的基礎和核心,一個組件包裝了一個特定的功能,並且組件之間協同工作以組裝成一個完整的應用程式。
一般來說,一個組件就是一個用於控制視圖範本的JavaScript類。
接下來我們在 angular-quickstart 創建一個 app 的目錄:
$ mkdir app
$ cd app
並添加組件檔 app.component.js ,內容如下:
app.component.js 檔:
(function(app) {
app.AppComponent =
ng.core.Component({
selector: 'my-app',
template: '<h1>我的第一個 Angular 應用</h1>'
})
.Class({
constructor: function() {}
});
})(window.app || (window.app = {}));
接下來我們來分析下以上代碼:
我們通過鏈式調用全局Angular core命名空間ng.core中的Component和Class方法創建了一個名為AppComponent的可視化組件。
Component方法接受一個包含兩個屬性的配置對象,Class方法是我們實現組件本身的地方,在Class方法中我們給組件添加屬性和方法,它們會綁定到相應的視圖和行為。
模組
Angular應用都是模組化的,ES5沒有內置的模組化系統,可以使用第三方模組系統,然後我們為應用創建獨立的命名空間 app,檔代碼可以包裹在 IIFE(立即執行函數運算式)中:
(function(app) {
})(window.app || (window.app = {}));
我們將全局app命名空間對象傳入IIFE中,如果不存在就用一個空對象初始化它。
大部分應用文件通過在app命名空間上添加東西來輸出代碼,我們在app.component.js檔中輸出了AppComponent。
app.AppComponent =
Class定義對象
本實例中AppComponent類只有一個空的構造函數:
.Class({
constructor: function() {}
});
當我們要創建一個是有實際意義的應用時,我們可以使用屬性和應用邏輯來擴展這個對象。
Component 定義對象
ng.core.Component()告訴Angular這個類定義對象是一個Angular組件。傳遞給ng.core.Component()的配置對象有兩個字段:selector和template。
ng.core.Component({
selector: 'my-app',
template: '<h1>我的第一個 Angular 應用</h1>'
})
selector 為一個宿主HTML元素定義了一個簡單的CSS選擇器my-app。當Angular在宿主HTML中遇到一個my-app元素時它創建並顯示一個AppComponent實例。
template 屬性容納著組件的範本。
添加 NgModule
Angular 應用由 Angular 模組組成,該模組包含了 Angular 應用所需要的組件及其他任何東西。
接下來我們創建 app/app.module.js 檔,內容如下:
app.module.js 檔:
(function(app) {
app.AppModule =
ng.core.NgModule({
imports: [ ng.platformBrowser.BrowserModule ],
declarations: [ app.AppComponent ],
bootstrap: [ app.AppComponent ]
})
.Class({
constructor: function() {}
});
})(window.app || (window.app = {}));
啟動應用
添加 app/main.js 檔:
app/main.js 檔:
(function(app) {
document.addEventListener('DOMContentLoaded', function() {
ng.platformBrowserDynamic
.platformBrowserDynamic()
.bootstrapModule(app.AppModule);
});
})(window.app || (window.app = {}));
我們需要兩樣東西來啟動應用:
- Angular 的 platformBrowserDynamic().bootstrapModule 函數。
-
上文中提到的應用根模組 AppModule。
接下來創建 index.html,代碼如下所示:
index.html 檔:
<html>
<head>
<meta charset="utf-8">
<title>Angular 2 實例 - IT研修(xuhuhu.com)</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="styles.css">
<script src="node_modules/core-js/client/shim.min.js"></script>
<script src="node_modules/zone.js/dist/zone.js"></script>
<script src="node_modules/reflect-metadata/Reflect.js"></script>
<script src="node_modules/rxjs/bundles/Rx.js"></script>
<script src="node_modules/@angular/core/bundles/core.umd.js"></script>
<script src="node_modules/@angular/common/bundles/common.umd.js"></script>
<script src="node_modules/@angular/compiler/bundles/compiler.umd.js"></script>
<script src="node_modules/@angular/platform-browser/bundles/platform-browser.umd.js"></script>
<script src="node_modules/@angular/platform-browser-dynamic/bundles/platform-browser-dynamic.umd.js"></script>
<script src='app/app.component.js'></script>
<script src='app/app.module.js'></script>
<script src='app/main.js'></script>
</head>
<body>
<my-app>Loading...</my-app>
</body>
</html>
index.html 分析
- 1、載入我們需要的JavaScript庫;
- 2、載入我們自己的JavaScript檔,注意順序;
- 3、我們在<body>標籤中添加<my-app>標籤。
執行過程為:當 Angular 在 main.js 中調用 bootstrapModule 函數時,它讀取 AppModule 的元數據,在啟動組件中找到 AppComponent 並找到 my-app 選擇器,定位到一個名字為 my-app 的元素,然後在這個標籤之間的載入內容。
添加一些樣式
styles.css 檔代碼為:
styles.css 檔:
h1 {
color: #369;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
body {
margin: 2em;
}
打開終端,輸入以下命令:
$ npm start
訪問 http://localhost:3000/,流覽器顯示結果為:
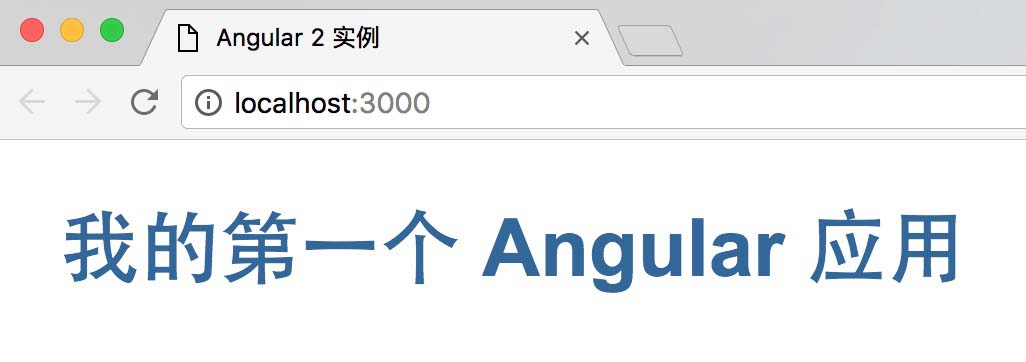
這樣我們的第一個 Angular2 的應用就算創建完成了,本文所使用的源碼可以通過以下方式下載,不包含 node_modules。
源代碼下載