Java 實例 - 獲取線程狀態
Java 線程的生命週期中,在 Thread 類裏有一個枚舉類型 State,定義了線程的幾種狀態,分別有:
- New
- Runnable
- Blocked
- Waiting
- Timed Waiting
- Terminated
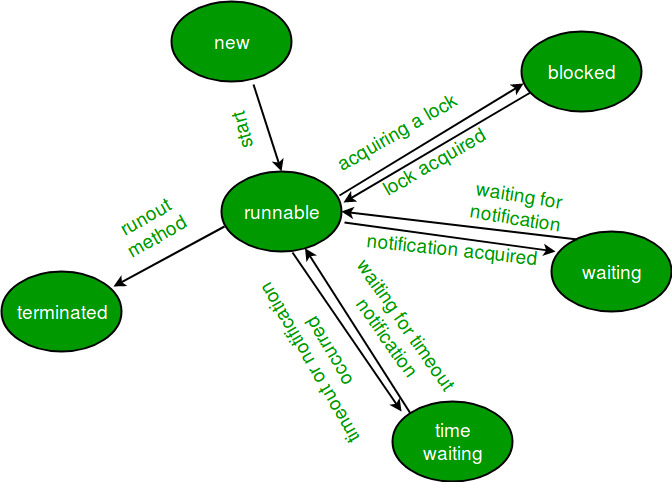
各個狀態說明:
1. 初始狀態 - NEW
聲明:
public static final Thread.State NEW
實現 Runnable 介面和繼承 Thread 可以得到一個線程類,new 一個實例出來,線程就進入了初始狀態。
2. RUNNABLE
聲明:
public static final Thread.State RUNNABLE
2.1. 就緒狀態
就緒狀態只是說你資格運行,調度程式沒有挑選到你,你就永遠是就緒狀態。
調用線程的 start() 方法,此線程進入就緒狀態。
當前線程 sleep() 方法結束,其他線程 join() 結束,等待用戶輸入完畢,某個線程拿到對象鎖,這些線程也將進入就緒狀態。
當前線程時間片用完了,調用當前線程的 yield() 方法,當前線程進入就緒狀態。
鎖池裏的線程拿到對象鎖後,進入就緒狀態。
2.2. 運行中狀態
線程調度程式從可運行池中選擇一個線程作為當前線程時線程所處的狀態。這也是線程進入運行狀態的唯一一種方式。
3. 阻塞狀態 - BLOCKED
聲明:
public static final Thread.State BLOCKED
阻塞狀態是線程阻塞在進入synchronized關鍵字修飾的方法或代碼塊(獲取鎖)時的狀態。
4. 等待 - WAITING
聲明:
public static final Thread.State WAITING
處於這種狀態的線程不會被分配 CPU 執行時間,它們要等待被顯式地喚醒,否則會處於無限期等待的狀態。
5. 超時等待 - TIMED_WAITING
聲明:
public static final Thread.State TIMED_WAITING
處於這種狀態的線程不會被分配 CPU 執行時間,不過無須無限期等待被其他線程顯示地喚醒,在達到一定時間後它們會自動喚醒。
6. 終止狀態 - TERMINATED
聲明:
public static final Thread.State TERMINATED
當線程的 run() 方法完成時,或者主線程的 main() 方法完成時,我們就認為它終止了。這個線程對象也許是活的,但是,它已經不是一個單獨執行的線程。線程一旦終止了,就不能複生。
在一個終止的線程上調用 start() 方法,會拋出 java.lang.IllegalThreadStateException 異常。
以下實例演示了如何獲取線程的狀態:
Main.java 檔
以上代碼運行輸出結果為:
State of thread1 after creating it - NEW State of thread1 after calling .start() method on it - RUNNABLE State of thread2 after creating it - NEW State of thread2 after calling .start() method on it - RUNNABLE State of thread2 after calling .sleep() method on it - TIMED_WAITING State of thread1 while it called join() method on thread2 -WAITING State of thread2 when it has finished it's execution - TERMINATED