链表基础知识
连接表是通过链接连接在一起的数据结构的一个序列。
链表是一个序列的链接,其中包含项目。每个链接中包含到另一条连接。链表是数组之后第二种最常用的数据结构。 以下是理解链表的概念,重要术语。
-
Link − 链表中的每个链路可以存储数据称为一个元素。
-
Next − 链表的每个链接包含一个链接到下一个被称为下一个。
-
LinkedList − LinkedList 包含连接链接到名为 First 的第一个环节。
链表表示
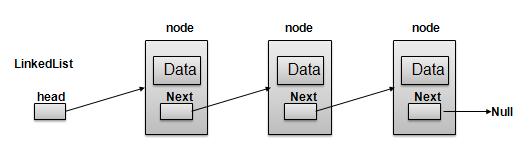
按照如上所示图中,以下是要考虑的重要问题。
- 链表包含一个名为第一个(first)的链接元素。
- 每个链路进行数据字段和链接域叫做下一个(next)。
- 每一个Link链接,其利用其下一个链接的下一个链接。
- 最后一个链接带有链接的空标记列表的末尾。
链表的类型
以下是链表的各种版本。
-
简单的链表 − 项目导航是向前。
-
双向链表 − 项目可以导航向前和向后的方式。
-
循环链表 − 最后一个项目中包含的第一个元素的链接在下一个以及第一个元素链接到最后一个元素的上一个。
基本操作
以下是通过一个列表支持的基本操作。
-
插入 − 在列表的开头添加元素。
-
删除 − 删除在列表开头的元素。
-
显示 − 显示完整列表。
-
搜索 − 搜索给定键的元素。
-
删除 − 删除给定键的元素。
插入操作
插入是三个步骤的过程 -
- 使用提供的数据创建一个新的连接。
- 指向新建链接到旧的第一个链接。
- 第一个链接指向到这个新链接。
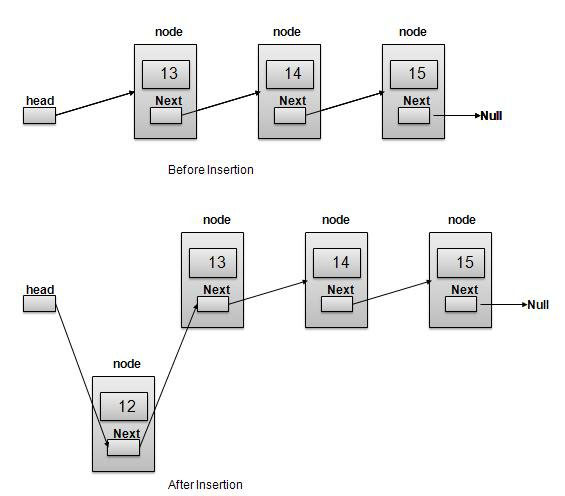
//insert link at the first location void insertFirst(int key, int data){ //create a link struct node *link = (struct node*) malloc(sizeof(struct node)); link->key = key; link->data = data; //point it to old first node link->next = head; //point first to new first node head = link; }
删除操作
删除是两个步骤过程-
- 找第一个链接指向作为临时链路相连。
- 第一个链接指向到临时链接的下一个链接。
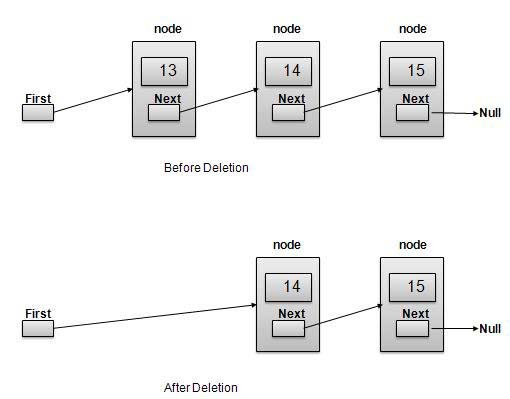
//delete first item struct node* deleteFirst(){ //save reference to first link struct node *tempLink = head; //mark next to first link as first head = head->next; //return the deleted link return tempLink; }
导航操作
导航是一个递归步骤的过程,是许多操作的基础,如:搜索,删除等 −
- 获取指向的第一个链接是当前链接的链接。
- 检查如果当前链接不为空则显示它。
- 指向当前链接到下一个链接,并移动到上面的步骤。
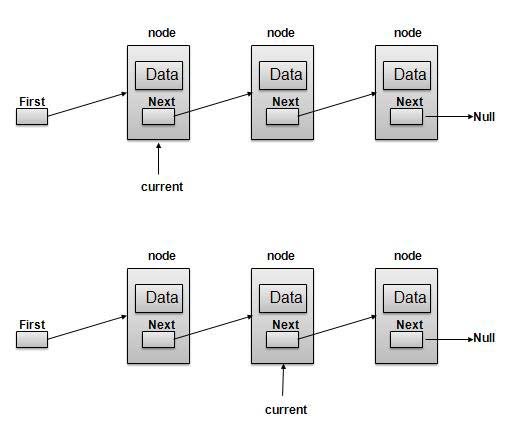
注意 −
//display the list void printList(){ struct node *ptr = head; printf("\n[ "); //start from the beginning while(ptr != NULL){ printf("(%d,%d) ",ptr->key,ptr->data); ptr = ptr->next; } printf(" ]"); }
高级操作
以下是一个列表中指定的高级操作
-
排序 − 排序基于一个特定列表上的顺序的
-
反转 − 反转链表
排序操作
我们使用冒泡排序排序列表。
void sort(){ int i, j, k, tempKey, tempData ; struct node *current; struct node *next; int size = length(); k = size ; for ( i = 0 ; i < size - 1 ; i++, k-- ) { current = head ; next = head->next ; for ( j = 1 ; j < k ; j++ ) { if ( current->data > next->data ) { tempData = current->data ; current->data = next->data; next->data = tempData ; tempKey = current->key; current->key = next->key; next->key = tempKey; } current = current->next; next = next->next; } } }
反转操作
下面的代码演示了逆转单向链表。
void reverse(struct node** head_ref) { struct node* prev = NULL; struct node* current = *head_ref; struct node* next; while (current != NULL) { next = current->next; current->next = prev; prev = current; current = next; } *head_ref = prev; }
要查看 C编程语言链表实现,请 点击 。
上一篇:
哈希表实例程序(C语言)
下一篇:
链表实例程序(C语言)