二叉搜索树表现出特殊的行为。一个节点的左子必须具备值小于它的父代值,并且节点的右子节点的值必须大于它的父值。
二叉搜索树表示
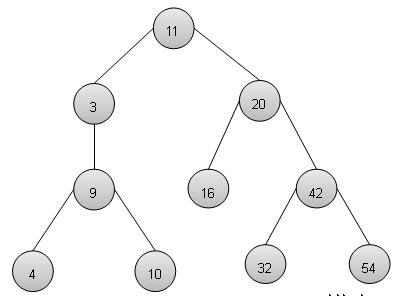
我们将使用节点对象来实现树,并通过引用连接它们。
基本操作
以下是遵循树的基本操作。
-
搜索 − 搜索一棵树中的元素。
-
插入 − 插入元素到一棵树中。
-
前序遍历 − 遍历一棵树方法。
-
后序遍历 − 遍历树在序方法。
-
后序遍历 − 遍历树的后序方法。
节点
限定了具有一些数据,引用其左,右子节点的节点。
struct node { int data; struct node *leftChild; struct node *rightChild; };
搜索操作
每当一个元素是被搜索。开始从根节点搜索,如果数据小于键值,在左子树中搜索元素,否则搜索元素在右子树。每一个节点按照同样的算法。
struct node* search(int data){ struct node *current = root; printf("Visiting elements: "); while(current->data != data){ if(current != NULL) { printf("%d ",current->data); //go to left tree if(current->data > data){ current = current->leftChild; }//else go to right tree else{ current = current->rightChild; } //not found if(current == NULL){ return NULL; } } } return current; }
插入操作
每当一个元素将被插入。首先找到它应有的位置。开始从根节点搜索,如果数据小于键值,搜索空位置在左子树那么插入数据。否则,搜索空位置在右子树并插入数据。
void insert(int data){ struct node *tempNode = (struct node*) malloc(sizeof(struct node)); struct node *current; struct node *parent; tempNode->data = data; tempNode->leftChild = NULL; tempNode->rightChild = NULL; //if tree is empty if(root == NULL){ root = tempNode; }else{ current = root; parent = NULL; while(1){ parent = current; //go to left of the tree if(data < parent->data){ current = current->leftChild; //insert to the left if(current == NULL){ parent->leftChild = tempNode; return; } }//go to right of the tree else{ current = current->rightChild; //insert to the right if(current == NULL){ parent->rightChild = tempNode; return; } } } } }